Overview
Katz Centrality measures the influence of a node by considering not only its immediate connections but also its indirect connections at various distances while diminishing importance to more distant nodes.
Katz centrality values range from 0 to 1, with higher scores indicating nodes that exert greater influence over the flow and connectivity of the network.
References:
- L. Katz, A New Status Index Derived from Sociometric Analysis (1953)
Concepts
Katz Centrality
The Katz centrality is an extension of the eigenvector centrality. In the k
-th round of influence propagation in eigenvector centrality, the centrality vector is simply updated as c(k) = Ac(k-1)
, where A
is the adjacency matrix. Katz centrality modifies this computation by introducing two additional parameters, leading to the following update formula (which should be rescaled afterward):
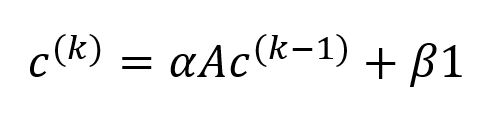
where,
α
(alpha) is an attenuation factor that controls the decay of influence over each propagation round. In thek
-th round, the influences from indirect neighbors that arek
steps away are considered, with their contributions cumulatively attenuated by a factor ofαk
. To ensure the convergence ofc(k)
,α
must be smaller than1/λmax
, where1/λmax
is the dominant eigenvalue of the adjacency matrixA
.β
(beta) is a baseline centrality constant that ensures every node has a nonzero centrality score, even if it receives no influence. The common choice forβ
is 1.1
is an n × 1 column vector of ones, where n is the number of nodes in the graph.

Example Graph
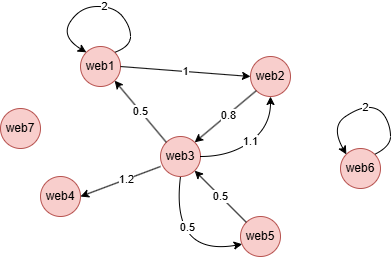
To create this graph:
// Runs each row separately in order in an empty graphset
create().node_schema("web").edge_schema("link")
create().edge_property(@link, "value", float)
insert().into(@web).nodes([{_id:"web1"}, {_id:"web2"}, {_id:"web3"}, {_id:"web4"}, {_id:"web5"}, {_id:"web6"}, {_id:"web7"}])
insert().into(@link).edges([{_from:"web1", _to:"web1",value:2}, {_from:"web1", _to:"web2",value:1}, {_from:"web2", _to:"web3",value:0.8}, {_from:"web3", _to:"web1",value:0.5}, {_from:"web3", _to:"web2",value:1.1}, {_from:"web3", _to:"web4",value:1.2}, {_from:"web3", _to:"web5",value:0.5}, {_from:"web5", _to:"web3",value:0.5}, {_from:"web6", _to:"web6",value:2}])
Creating HDC Graph
To load the entire graph to the HDC server hdc-server-1
as hdc_katz
:
CALL hdc.graph.create("hdc-server-1", "hdc_katz", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
})
hdc.graph.create("hdc_katz", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
Parameters
Algorithm name: katz_centrality
Name |
Type |
Spec |
Default |
Optional |
Description |
---|---|---|---|---|---|
max_loop_num |
Integer | ≥1 | 20 |
Yes | The maximum number of iteration rounds. The algorithm will terminate after completing all rounds. |
tolerance |
Float | (0,1) | 0.001 |
Yes | The algorithm terminates when the changes in all scores between iterations are less than the specified tolerance , indicating that the result is stable. |
edge_weight_property |
"<@schema.?><property> " |
/ | / | Yes | A numeric edge property used as weights in the adjacency matrix A ; edges without this property are ignored. |
direction |
String | in , out |
/ | Yes | Constructs the adjacency matrix A with the in-links (in ) or out-links (out ) of each node. |
alpha |
Float | (0, 1/λmax) | 0.25 |
Yes | The attenuation factor, which must be less than the inverse of dominant eigenvalue (λmax) of the adjacency matrix A . |
beta |
Float | >0 | 1 |
Yes | The baseline centrality constant that ensures every node has a nonzero centrality score. |
return_id_uuid |
String | uuid , id , both |
uuid |
Yes | Includes _uuid , _id , or both to represent nodes in the results. |
limit |
Integer | ≥-1 | -1 |
Yes | Limits the number of results returned; -1 includes all results. |
order |
String | asc , desc |
/ | Yes | Sorts the results by katz_centrality . |
File Writeback
CALL algo.katz_centrality.write("hdc_katz", {
params: {
return_id_uuid: "id",
max_loop_num: 15,
tolerance: 0.01,
direction: "in"
},
return_params: {
file: {
filename: "katz_centrality"
}
}
})
algo(katz_centrality).params({
projection: "hdc_katz",
return_id_uuid: "id",
max_loop_num: 15,
tolerance: 0.01,
direction: "in"
}).write({
file: {
filename: "katz_centrality"
}
})
Result:
_id,katz_centrality
web3,0.399527
web7,0.335089
web1,0.40142
web5,0.368555
web6,0.365776
web2,0.401707
web4,0.368732
DB Writeback
Writes the katz_centrality
values from the results to the specified node property. The property type is double
.
CALL algo.katz_centrality.write("hdc_katz", {
params: {
edge_weight_property: "@link.value"
},
return_params: {
db: {
property: "kc"
}
}
})
algo(katz_centrality).params({
projection: "hdc_katz",
edge_weight_property: "@link.value"
}).write({
db:{
property: 'kc'
}
})
Full Return
CALL algo.katz_centrality("hdc_katz", {
params: {
return_id_uuid: "id",
max_loop_num: 20,
tolerance: 0.01,
edge_weight_property: "value",
direction: "in",
alpha: 0.25,
beta: 1,
order: 'desc'
},
return_params: {}
}) YIELD kc
RETURN kc
exec{
algo(katz_centrality).params({
return_id_uuid: "id",
max_loop_num: 20,
tolerance: 0.01,
edge_weight_property: "value",
direction: "in",
alpha: 0.25,
beta: 1,
order: 'desc'
}) as kc
return kc
} on hdc_katz
Result:
_id | katz_centrality |
---|---|
web1 | 0.416245 |
web2 | 0.400671 |
web6 | 0.398739 |
web3 | 0.373287 |
web4 | 0.369527 |
web5 | 0.347756 |
web7 | 0.332239 |
Stream Return
CALL algo.katz_centrality("hdc_katz", {
params: {
edge_weight_property: "@link.value",
direction: "in"
},
return_params: {
stream: {}
}
}) YIELD kc
RETURN CASE
WHEN kc.katz_centrality > 0.4 THEN "important"
ELSE "normal"
END as r, count(r) GROUP BY r
exec{
algo(katz_centrality).params({
edge_weight_property: "@link.value",
direction: "in"
}).stream() as kc
with case
when kc.katz_centrality > 0.4 then "important"
else "normal"
end as r
group by r
return r, count(r)
} on hdc_katz
Result:
r | count(r) |
---|---|
important | 2 |
normal | 5 |