Overview
The Schema Overview algorithm summarizes the structure of a graph by presenting the statistics of the source node schema (label), edge schema, end node schema, and the count of edges.
Example Graph
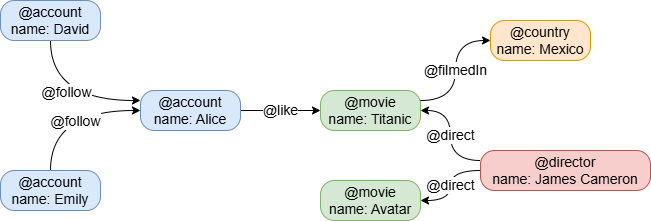
To create this graph:
// Runs each row separately in order in an empty graphset
create().node_schema("account").node_schema("movie").node_schema("country").node_schema("director").edge_schema("follow").edge_schema("like").edge_schema("filmedIn").edge_schema("direct")
insert().into(@account).nodes([{_id:"David"}, {_id:"Emily"}, {_id:"Alice"}])
insert().into(@movie).nodes([{_id:"Titanic"}, {_id:"Avatar"}])
insert().into(@country).nodes({_id:"Mexico"})
insert().into(@director).nodes({_id:"James Cameron"})
insert().into(@follow).edges([{_from:"David", _to:"Alice"}, {_from:"Emily", _to:"Alice"}])
insert().into(@like).edges({_from:"Alice", _to:"Titanic"})
insert().into(@filmedIn).edges({_from:"Titanic", _to: "Mexico"})
insert().into(@direct).edges([{_from:"James Cameron", _to:"Titanic"}, {_from:"James Cameron", _to:"Avatar"}])
Creating HDC Graph
To load the entire graph to the HDC server hdc-server-1
as hdc_schema_ov
:
CALL hdc.graph.create("hdc-server-1", "hdc_schema_ov", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
})
hdc.graph.create("hdc_schema_ov", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
Parameters
Algorithm name: schema_overview
Name |
Type |
Spec |
Default |
Optional |
Description |
---|---|---|---|---|---|
order |
String | asc , desc |
/ | Yes | Sorts the results by count . |
Full Return
CALL algo.schema_overview("hdc_schema_ov", {
params: {},
return_params: {}
}) YIELD result
RETURN result
exec{
algo(schema_overview).params() as result
return result
} on hdc_schema_ov
Result:
node schema(src) |
edge schema |
node schema(dest) |
count |
---|---|---|---|
account | follow | account | 2 |
account | like | movie | 1 |
movie | filmedIn | country | 1 |
director | direct | movie | 2 |
Stream Return
CALL algo.schema_overview("hdc_schema_ov", {
params: {},
return_params: {
stream: {}
}
}) YIELD r
FILTER r.schema = "account"
RETURN r
exec{
algo(schema_overview).params().stream() as r
where r.`node schema(src)` == "account"
return r
} on hdc_schema_ov
Result:
node schema(src) |
edge schema |
node schema(dest) |
count |
---|---|---|---|
account | follow | account | 2 |
account | like | movie | 1 |