Overview
The Total Neighbors algorithm computes the total number of distinct neighbors of two nodes as a measure of their similarity.
This algorithm takes into account the entire neighborhood of both nodes, giving a more comprehensive view of their similarity compared to algorithms that only focus on common neighbors. It is computed using the following formula:

where N(x) and N(y) are the sets of adjacent nodes to nodes x and y respectively.
More total neighbors indicate greater similarity between nodes, while a number of 0 indicates no similarity between two nodes.
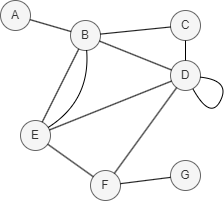
In this example, TN(D,E) = |N(D) ∪ N(E)| = |{B, C, E, F} ∪ {B, D, F}| = |{B, C, D, E, F}| = 5.
Considerations
- The Total Neighbors algorithm ignores the direction of edges but calculates them as undirected edges.
Example Graph
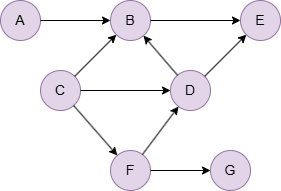
To create this graph:
// Runs each row separately in order in an empty graphset
insert().into(@default).nodes([{_id:"A"}, {_id:"B"}, {_id:"C"}, {_id:"D"}, {_id:"E"}, {_id:"F"}, {_id:"G"}])
insert().into(@default).edges([{_from:"A", _to:"B"}, {_from:"B", _to:"E"}, {_from:"C", _to:"B"}, {_from:"C", _to:"D"}, {_from:"C", _to:"F"}, {_from:"D", _to:"B"}, {_from:"D", _to:"E"}, {_from:"F", _to:"D"}, {_from:"F", _to:"G"}])
Creating HDC Graph
To load the entire graph to the HDC server hdc-server-1
as hdc_tlp
:
CALL hdc.graph.create("hdc-server-1", "hdc_tlp", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
})
hdc.graph.create("hdc_tlp", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
Parameters
Algorithm name: topological_link_prediction
Name |
Type |
Spec |
Default |
Optional |
Description |
---|---|---|---|---|---|
ids |
[]_id |
/ | / | No | Specifies the first group of nodes for computation by their _id ; computes for all nodes if it is unset. |
uuids |
[]_uuid |
/ | / | No | Specifies the first group of nodes for computation by their _uuid ; computes for all nodes if it is unset. |
ids2 |
[]_id |
/ | / | No | Specifies the second group of nodes for computation by their _id ; computes for all nodes if it is unset. |
uuids2 |
[]_uuid |
/ | / | No | Specifies the second group of nodes for computation by their _uuid ; computes for all nodes if it is unset. |
type |
String | Total_Neighbors |
Adamic_Adar |
No | Specifies the similarity type; for Total Neighbors, keep it as Total_Neighbors . |
return_id_uuid |
String | uuid , id , both |
uuid |
Yes | Includes _uuid , _id , or both to represent nodes in the results. |
limit |
Integer | ≥-1 | -1 |
Yes | Limits the number of results returned; -1 includes all results. |
File Writeback
CALL algo.topological_link_prediction.write("hdc_tlp", {
params: {
ids: ["C"],
ids2: ["A","E","G"],
type: "Total_Neighbors",
return_id_uuid: "id"
},
return_params: {
file: {
filename: "tn.txt"
}
}
})
algo(topological_link_prediction).params({
projection: "hdc_tlp",
ids: ["C"],
ids2: ["A","E","G"],
type: "Total_Neighbors",
return_id_uuid: "id"
}).write({
file: {
filename: "tn.txt"
}
})
Result:
_id1,_id2,result
C,A,3
C,E,3
C,G,3
Full Return
CALL algo.topological_link_prediction("hdc_tlp", {
params: {
ids: ["C"],
ids2: ["A","C","E","G"],
type: "Total_Neighbors",
return_id_uuid: "id"
},
return_params: {}
}) YIELD tn
RETURN tn
exec{
algo(topological_link_prediction).params({
ids: ["C"],
ids2: ["A","C","E","G"],
type: "Total_Neighbors",
return_id_uuid: "id"
}) as tn
return tn
} on hdc_tlp
Result:
_id1 | _id2 | result |
---|---|---|
C | A | 3 |
C | E | 3 |
C | G | 3 |
Stream Return
MATCH (n)
RETURN collect_list(n._id) AS IdList
NEXT
CALL algo.topological_link_prediction("hdc_tlp", {
params: {
ids: ["C"],
ids2: IdList,
type: "Total_Neighbors",
return_id_uuid: "id"
},
return_params: {
stream: {}
}
}) YIELD tn
FILTER tn.result >= 4
RETURN tn
find().nodes() as n
with collect(n._id) as IdList
exec{
algo(topological_link_prediction).params({
ids: ["C"],
ids2: IdList,
type: "Total_Neighbors",
return_id_uuid: "id"
}).stream() as tn
where tn.result >= 4
return tn
} on hdc_tlp
Result:
_id1 | _id2 | result |
---|---|---|
C | B | 6 |
C | D | 5 |
C | F | 5 |