Overview
To execute a UQL query on an HDC graph, use the syntax:
exec{
<query>
} on <hdcGraphName>
HDC graphs support queries that retrieve data from the database with enhanced efficiency. However, remember that any query attempting to write data will fail on HDC graphs.
Example Graph
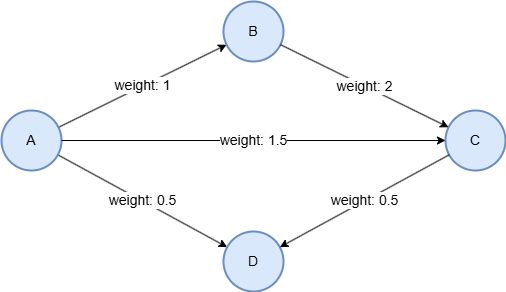
To create the graph, execute each of the following UQL queries sequentially in an empty graphset:
create().node_schema("entity").edge_schema("link")
create().edge_property(@link, "weight", float)
insert().into(@entity).nodes([{_id:"A"},{_id:"B"},{_id:"C"},{_id:"D"}])
insert().into(@link).edges([{_from:"A", _to:"B", weight:1},{_from:"A", _to:"C", weight:1.5},{_from:"A", _to:"D", weight:0.5},{_from:"B", _to:"C", weight:2},{_from:"C", _to:"D", weight:0.5}])
Queries on HDC Graphs
To create an HDC graph hdcGraph
of the entire graph:
hdc.graph.create("hdcGraph", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
To run the ab()
query on hdcGraph
:
exec{
ab().src({_id == "A"}).dest({_id == "C"}).depth(2) as p
return p
} on hdcGraph
Result: p
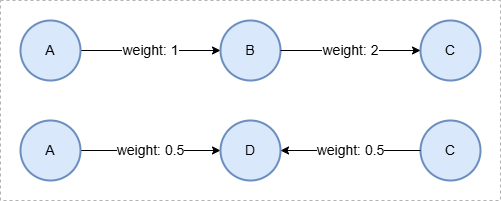
To run the khop()
query on hdcGraph
:
exec{
khop().src({_id == "A"}).depth(1).direction(right) as n
return count(n)
} on hdcGraph
Result:
count(n) |
---|
3 |
Limited Graph Traversal Direction
If an HDC graph is created with the direction
option set to in
or out
, graph traversal is restricted to incoming or outgoing edges, respectively. Queries attempting to traverse in the missing direction throws errors or yields empty results.
To create an HDC graph hdcGraph_in_edges
of the graph with nodes and incoming edges:
hdc.graph.create("hdcGraph_in_edges", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "in",
load_id: true,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
This khop()
query attempts to follow the outgoing (right) edges on hdcGraph_in_edges
, no result will be returned.
exec{
khop().src({_id == "A"}).depth(1).direction(right) as n
return count(n)
} on hdcGraph_in_edges
Result:
count(n) |
---|
0 |
Exclusion of Node IDs
If an HDC graph is created with the load_id
option set to false
, it does not contain the _id
values for nodes. Queries referencing _id
throws errors or yields empty results.
To create an HDC graph hdcGraph_no_id
of the graph without nodes' _id
values:
hdc.graph.create("hdcGraph_no_id", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: false,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
The ab()
query utilizes _id
to filter nodes on hdcGraph_no_id
, error occurs as the HDC graph lacks nodes' _id
:
exec{
ab().src({_id == "A"}).dest({_id == "C"}).depth(2) as p
return p{*}
} on hdcGraph_no_id
Exclusion of Properties
If an HDC graph is created without certain properties, queries referencing those properties throws errors or yields empty results.
To create an HDC graph hdcGraph_no_weight
of the graph containing nodes and only system properties of @link
edges:
hdc.graph.create("hdcGraph_no_weight", {
nodes: {"*": ["*"]},
edges: {"link": []},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
The ab()
query finds shortest path between two nodes on hdcGraph_no_weight
, weighted by the edge property @link.weight
, error occurs as the weight
property is missing:
exec{
ab().src({_id == "A"}).dest({_id == "C"}).depth(2).shortest(@link.weight) as p
return p
} on hdcGraph_no_weight
Unsupported Data Modifications
The following query results in an error indicating that node insertion is not supported on HDC graphs:
exec{
insert().into(@entity).nodes([{_id: "E"}])
} on hdcGraph