Overview
The insert()
statement allows for the insertion of new nodes and edges to a single schema in a graphset.
// Inserts nodes
insert().into(<schema>).nodes([
{<property1?>: <value1?>, <property2?>: <value2?>, ...},
{<property1?>: <value1?>, <property2?>: <value2?>, ...},
...
])
// Inserts edges
insert().into(<schema>).edges([
{_from: <fromValue>, _to: <toValue>, <property1?>: <value1?>, <property2?>: <value2?>, ...},
{_from: <fromValue>, _to: <toValue>, <property1?>: <value1?>, <property2?>: <value2?>, ...},
...
])
Method |
Param |
Description |
---|---|---|
into() |
<schema> |
Specifies the node or edge schema (e.g., @user ). |
nodes() or edges() |
List of property specifications | Allows the insertion of one or more nodes or edges into the specified schema, with each property specification wrapped in {} . |
In the property specifications, each provided property will be assigned its given value, while any missing custom properties will default to null
.
Please note:
- Node
_id
values will be generated by the system if not provided, though custom unique values can be assigned if desired. - Node and edge
_uuid
values are always generated by the system and cannot be manually assigned. - Edge properties
_from
and_to
must be provided to specify the source and destination nodes of the edge.
Example Graph
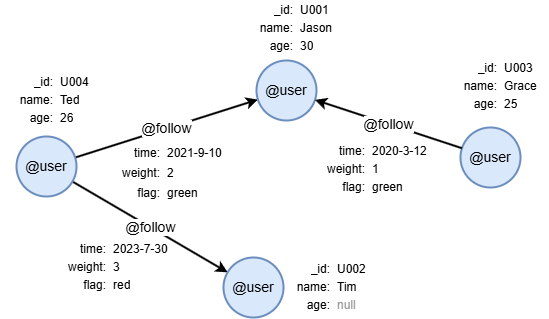
To create the graph, execute each of the following UQL queries sequentially in an empty graphset:
create().node_schema("user").edge_schema("follow")
create().node_property(@user, "name").node_property(@user, "age", int32).node_property(@user, "location", point).node_property(@user, "interests", "string[]").edge_property(@follow, "time", datetime)
insert().into(@user).nodes([{_id:"U001", name:"Jason", age:30}, {_id:"U002", name:"Tim"}, {_id:"U003", name:"Grace", age:25}, {_id:"U004", name:"Ted", age:26}])
insert().into(@follow).edges([{_from:"U004", _to:"U001", time:"2021-9-10"}, {_from:"U003", _to:"U001", time:"2020-3-12"}, {_from:"U004", _to:"U002", time:"2023-7-30"}])
Inserting a Single Node/Edge
To insert a node into @user
:
insert().into(@user).nodes({_id: "U005", name: "Alice"})
To insert an edge into @follow
:
insert().into(@follow).edges({_from: "U002", _to: "U001", time: "2023-8-9"})
When inserting a single node or edge, the outer brackets
[]
innodes()
oredges()
can be omitted.
Inserting Multiple Nodes/Edges
To insert three nodes into @user
:
insert().into(@user).nodes([
{_id: "U006", name: "Lee", age: 12},
{name: "Alex"},
{}
])
To insert two edges into @follow
:
insert().into(@follow).edges([
{_from: "U001", _to: "U002"},
{_from: "U004", _to: "U003", time: "2023-9-10"}
])
Returning Inserted Data
To insert two edges into @follow
and return all the information of the inserted edges:
insert().into(@follow).edges([
{_from: "U001", _to: "U004"},
{_from: "U001", _to: "U003", time: "2022-2-7"}
]) as e
return e{*}
Result: e
_uuid |
_from |
_to |
_from_uuid |
_to_uuid |
schema |
values |
---|---|---|---|---|---|---|
Sys-gen | U001 | U004 | UUID of U001 | UUID of U004 | follow | {time: null } |
Sys-gen | U001 | U003 | UUID of U001 | UUID of U003 | follow | {time: "2022-02-07 00:00:00"} |
Providing Point-Type Values
You need to use the point()
function to specify the value of a point
-type property.
To insert a node into @user
while providing the value for the property location
of the point
type:
insert().into(@user).nodes([
{location: point({latitude: 132.1, longitude: -1.5})}
]) as n
return n.location
Result:
n.location |
---|
POINT(132.1 -1.5) |
Providing List-Type Values
To insert a node into @user
while providing the value for the property interests
of the string[]
type:
insert().into(@user).nodes([
{interests: ["violin", "tennis", "movie"]}
])