Overview
The upsert()
statement allows for (1) the update of existing nodes and edges, or (2) the insertion of new nodes and edges within a single schema in a graphset.
// Updates or inserts nodes
upsert().into(@<schema>).nodes([
{<property1?>: <value1?>, <property2?>: <value2?>, ...},
{<property1?>: <value1?>, <property2?>: <value2?>, ...},
...
])
// Updates or inserts edges
upsert().into(@<schema>).edges([
{_from: <fromValue>, _to: <toValue>, <property1?>: <value1?>, <property2?>: <value2?>, ...},
{_from: <fromValue>, _to: <toValue>, <property1?>: <value1?>, <property2?>: <value2?>, ...},
...
])
Method |
Param |
Description |
---|---|---|
into() |
<schema> |
Specifies the node or edge schema (e.g., @user ). |
nodes() or edges() |
List of property specifications | Allows the update or insertion of one or more nodes or edges into the specified schema, with each property specification wrapped in {} . |
Update
Node update occurs when an existing _id
value is provided in the property specification. Edge update occurs when an existing EDGE KEY constraint value is specified along with the corresponding _from
and _to
values.
When a node or edge is updated:
- Provided custom property values replace existing ones.
- Missing custom properties remain unchanged.
- System properties remain unchanged.
Insertion
A new node is inserted when a new _id
value is provided or when it is missing. A new edge is inserted when an EDGE KEY constraint is defined and a new EDGE KEY constraint value is provided along with _from
and _to
.
When a node or edge is inserted:
- Provided properties are assigned the given values.
- Missing custom properties default to
null
. - Node
_id
is generated by the system if not provided. Node or edge_uuid
is always generated by the system and cannot be manually assigned.
Example Graph
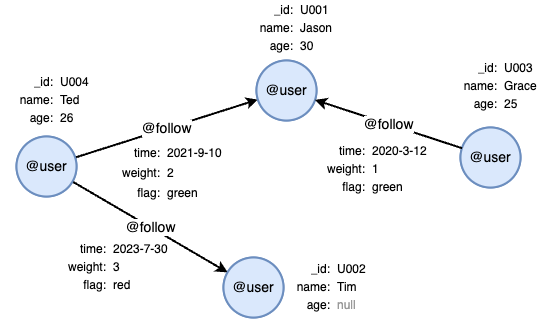
To create the graph, execute each of the following UQL queries sequentially in an empty graphset:
create().node_schema("user").edge_schema("follow")
create().node_property(@user, "name").node_property(@user, "age", int32).edge_property(@*, "time", datetime).edge_property(@*, "weight", int32).edge_property(@*, "flag")
insert().into(@user).nodes([{_id:"U001", name:"Jason", age:30}, {_id:"U002", name:"Tim"}, {_id:"U003", name:"Grace", age:25}, {_id:"U004", name:"Ted", age:26}])
insert().into(@follow).edges([{_from:"U004", _to:"U001", time:"2021-9-10", weight:2, flag: "green"}, {_from:"U003", _to:"U001", time:"2020-3-12", weight:1, flag: "green"}, {_from:"U004", _to:"U002", time:"2023-7-30", weight:3, flag: "red"}])
Updating or Inserting Nodes
To update or insert nodes into @user
:
upsert().into(@user).nodes([
{_id: "U001", name: "John"},
{_id: "U005", name: "Alice"},
{age: 12}
]) as n
return n{*}
- First node: The provided
_id
U001 already exists in the graph, so the corresponding node is updated. - Second node: The provided
_id
U005 is new, so a new node is inserted. - Third node:
_id
is not provided, so a new node is inserted.
Result: n
_id |
_uuid |
schema |
values |
---|---|---|---|
U001 | Sys-gen | user | {name: "John", age: 30} |
U005 | Sys-gen | user | {name: "Alice", age: null } |
Sys-gen | Sys-gen | user | {name: null , age: 12} |
Updating or Inserting Edges
No EDGE KEY Constraint
When there is no EDGE KEY constraint defined, this query throws an error:
upsert().into(@follow).edges([
{_from: "U004", _to: "U001", time: "2021-9-10"},
{_from: "U001", _to: "U004", time: "2021-10-3", weight: 2}
])
Single-Property EDGE KEY Constraint
To create a single-property EDGE KEY constraint on the edge property time
:
CREATE CONSTRAINT key_time
FOR ()-[e]-() REQUIRE e.time IS EDGE KEY
OPTIONS {
type: {time: "datetime"}
}
To update or insert edges into @follow
:
upsert().into(@follow).edges([
{_from: "U004", _to: "U001", time: "2021-9-10"},
{_from: "U001", _to: "U004", time: "2021-10-3", weight: 2}
]) as e
return e{*}
- First edge: The provided EDGE KEY constraint (
time
) with the value2021-9-10
already exists in the graph, and both_from
and_to
match, so the corresponding edge is updated. - Second edge: The provided EDGE KEY constraint (
time
) with the value2021-10-3
is new, so a new edge is inserted.
Result: e
_uuid |
_from |
_to |
_from_uuid |
_to_uuid |
schema |
values |
---|---|---|---|---|---|---|
Sys-gen | U004 | U001 | UUID of U004 | UUID of U001 | follow | {time: "2021-09-10 00:00:00", weight: 2, flag: "green"} |
Sys-gen | U001 | U002 | UUID of U001 | UUID of U002 | follow | {time: "2021-10-03 00:00:00", weight: 2, flag: null } |
Composite EDGE KEY Constraint
To create a composite EDGE KEY constraint on the edge properties time
and weight
:
CREATE CONSTRAINT key_time_weight
FOR ()-[e]-() REQUIRE (e.time, e.weight) IS EDGE KEY
OPTIONS {
type: {time: "datetime", weight: "int32"}
}
To update or insert edges into @follow
:
upsert().into(@follow).edges([
{_from: "U004", _to: "U001", time: "2021-9-10", weight: 2},
{_from: "U003", _to: "U001", time: "2020-3-12", weight: 2},
{_from: "U001", _to: "U004", time: "2021-10-3", weight: 1, flag: "green"}
]) as e
return e{*}
- First edge: The provided EDGE KEY constraint (
time
,weight
) with the combined value (2021-9-10
,2
) already exists in the graph, and both_from
and_to
match, so the corresponding edge is updated. - Second edge: The provided EDGE KEY constraint (
time
,weight
) with the combined value (2020-3-12
,2
) is new, so a new edge is inserted. - Third edge: The provided EDGE KEY constraint (
time
,weight
) with the combined value (2021-10-3
,1
) is new, so a new edge is inserted.
Result: e
_uuid |
_from |
_to |
_from_uuid |
_to_uuid |
schema |
values |
---|---|---|---|---|---|---|
Sys-gen | U004 | U001 | UUID of U004 | UUID of U001 | follow | {time: "2021-09-10 00:00:00", weight: 2, flag: "green"} |
Sys-gen | U003 | U001 | UUID of U003 | UUID of U001 | follow | {time: "2020-03-12 00:00:00", weight: 2, flag: null } |
Sys-gen | U001 | U004 | UUID of U001 | UUID of U004 | follow | {time: "2021-10-03 00:00:00", weight: 1, flag: "green"} |