Overview
The INSERT
statement allows you to add new nodes and edges into the graph using path patterns. Before inserting data, ensure that the required node and edge schemas (labels) and properties are already defined.
Example Graph
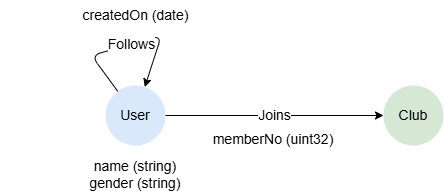
The following examples rely on a graph of the above structure. To create a graph with this structure:
CREATE GRAPH myGraph {
NODE User ({name string, gender string}),
NODE Club (),
EDGE Follows ()-[{createdOn date}]->(),
EDGE Joins ()-[{memberNo uint32}]->()
} PARTITION BY HASH(Crc32) SHARDS [1]
Inserting Nodes
Nodes can be inserted using node patterns:
- Each node must be assigned a label and may optionally include property specification. Missing property values default to
null
. - Node
_id
values will be generated by the system if not provided, though custom unique values can be assigned if desired. - Node
_uuid
values are always generated by the system and cannot be manually assigned.
To insert three nodes, one User
node with _id
and name
specified, one Club
node with only _id
specified, and one Club
node with no property specified:
INSERT (:User {_id: "U01", name: 'Quasar92'}), (:Club {_id: "C01"}), (:Club)
To insert and return one node:
INSERT (mochaeach:User {_id: "U02", name: 'mochaeach', gender: 'female'})
RETURN mochaeach
Result: mochaeach
_id | _uuid | schema | values |
---|---|---|---|
U02 | Sys-gen | User | {name: "mochaeach", gender: "female"} |
Inserting Edges
Edges can be inserted using path patterns where the edge pattern has node patterns on both sides and must include a direction to indicate its source and destination nodes:
- Each edge must be assigned a label and may optionally include property specification. Missing property values default to
null
. - Edge
_uuid
values are always generated by the system and cannot be manually assigned. - Edge
_from
,_to
,_from_uuid
, and_to_uuid
values are automatically derived from the specified source and destination nodes. They cannot be manually assigned.
When inserting edges, you must either connect them to existing nodes in the graph or insert the source and destination nodes along with the edges.
Inserting Nodes Along with Edges
To insert three User
nodes and two Follows
edges between them:
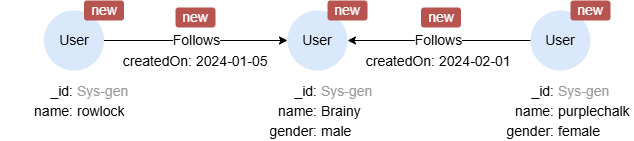
INSERT (:User {name: 'rowlock'})-[:Follows {createdOn: '2024-01-05'}]->(:User {name: 'Brainy', gender: 'male'})<-[:Follows {createdOn: '2024-02-01'}]-(:User {name: 'purplechalk', gender: 'female'})
Connecting to Existing Nodes
To insert and return a Joins
edge, with its source and destination nodes being found by the preceding MATCH
statement and bound to variables n1
and n2
:
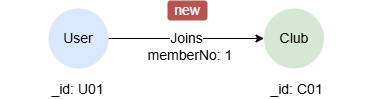
MATCH (n1:User {_id: 'U01'}), (n2:Club {_id: 'C01'})
INSERT (n1)-[e:Joins {memberNo: 1}]->(n2)
RETURN e
Result: e
_uuid |
_from |
_to |
_from_uuid |
_to_uuid |
schema |
values |
---|---|---|---|---|---|---|
Sys-gen | U01 | C01 | UUID of U01 | UUID of C01 | Joins | {memberNo: 1} |
Inserting Connected Paths
To insert two paths that intersect at a node by reusing the variable c02
:
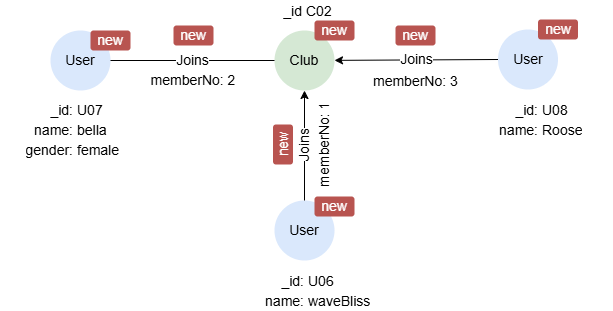
INSERT (:User {_id: 'U06', name: 'waveBliss'})-[:Joins {memberNo: 1}]->(c02:Club {_id: 'C02'})<-[:Joins {memberNo: 2}]-(:User {_id: 'U07', name: 'bella', gender: 'female'}),
(:User {_id: 'U08', name: 'Roose'})-[:Joins {memberNo: 3}]->(c02)