Overview
The Struc2Vec Walk is a biased random walk. This is one of the crucial componments of the Struc2Vec framework, where the walk is performed in a constructed multilayer weighted graph rather than the original graph. Please refer to the Struc2Vec algorithm for the details.
Example Graph
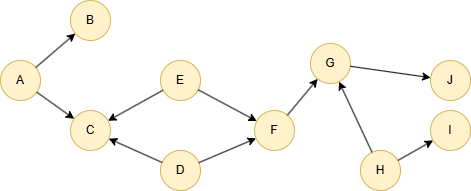
To create this graph:
// Runs each row separately in order in an empty graphset
insert().into(@default).nodes([{_id:"A"},{_id:"B"},{_id:"C"},{_id:"D"},{_id:"E"},{_id:"F"},{_id:"G"},{_id:"H"},{_id:"I"},{_id:"J"}])
insert().into(@default).edges([{_from:"A", _to:"B"}, {_from:"A", _to:"C"}, {_from:"D", _to:"C"}, {_from:"D", _to:"F"}, {_from:"E", _to:"C"}, {_from:"E", _to:"F"}, {_from:"F", _to:"G"}, {_from:"G", _to:"J"}, {_from:"H", _to:"G"}, {_from:"H", _to:"I"}])
Creating HDC Graph
To load the entire graph to the HDC server hdc-server-1
as hdc_struc2vec_walk
:
CALL hdc.graph.create("hdc-server-1", "hdc_struc2vec_walk", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
})
hdc.graph.create("hdc_struc2vec_walk", {
nodes: {"*": ["*"]},
edges: {"*": ["*"]},
direction: "undirected",
load_id: true,
update: "static",
query: "query",
default: false
}).to("hdc-server-1")
Parameters
Algorithm name: random_walk_struc2vec
Name |
Type |
Spec |
Default |
Optional |
Description |
---|---|---|---|---|---|
ids |
[]_id |
/ | / | Yes | Specifies nodes to start random walk by their _id ; computes for all nodes if it is unset. |
uuids |
[]_uuid |
/ | / | Yes | Specifies nodes to start random walk by their _uuid ; computes for all nodes if it is unset. |
walk_length |
Integer | ≥1 | 1 |
Yes | Depth of each walk, i.e., the number of nodes to visit. |
walk_num |
Integer | ≥1 | 1 |
Yes | Number of walks to perform for each specified node. |
k |
Integer | [1, 10] | / | No | Number of layers in the constructed multilayer weighted graph, which should not exceed the diameter of the original graph. |
stay_probability |
Float | (0,1] | / | No | The probability of walking in the current level. |
return_id_uuid |
String | uuid , id , both |
uuid |
Yes | Includes _uuid , _id , or both values to represent nodes in the results. |
limit |
Integer | ≥-1 | -1 |
Yes | Limits the number of results returned; -1 includes all results. |
File Writeback
CALL algo.random_walk_struc2vec.write("hdc_struc2vec_walk", {
params: {
return_id_uuid: "id",
walk_length: 5,
walk_num: 1,
k: 4,
stay_probability: 0.8
},
return_params: {
file: {
filename: "walks"
}
}
})
algo(random_walk_struc2vec).params({
project: "hdc_struc2vec_walk",
return_id_uuid: "id",
walk_length: 5,
walk_num: 1,
k: 4,
stay_probability: 0.8
}).write({
file:{
filename: 'walks'
}})
Result:
_ids
J,G,F,E,C,
D,F,E,F,E,
F,G,F,
H,I,H,F,
B,A,B,A,B,
A,C,E,D,
E,F,G,H,G,
C,D,F,G,
I,H,G,F,E,
G,D,F,
Full Return
CALL algo.random_walk_struc2vec("hdc_struc2vec_walk", {
params: {
return_id_uuid: "id",
ids: ['J'],
walk_length: 6,
walk_num: 3,
k: 4,
stay_probability: 0.8
},
return_params: {}
}) YIELD walks
RETURN walks
exec{
algo(random_walk_struc2vec).params({
return_id_uuid: "id",
ids: ['J'],
walk_length: 6,
walk_num: 3,
k: 4,
stay_probability: 0.8
}) as walks
return walks
} on hdc_struc2vec_walk
Result:
_ids |
---|
["J","G","F","C","B"] |
["J","F","H","F","J"] |
["J","G","J","H","F"] |
Stream Return
CALL algo.random_walk_struc2vec("hdc_struc2vec_walk", {
params: {
return_id_uuid: "id",
ids: ['J'],
walk_length: 6,
walk_num: 3,
k: 5,
stay_probability: 0.7
},
return_params: {
stream: {}
}
}) YIELD walks
RETURN walks
exec{
algo(random_walk_struc2vec).params({
return_id_uuid: "id",
ids: ['J'],
walk_length: 6,
walk_num: 3,
k: 5,
stay_probability: 0.7
}).stream() as walks
return walks
} on hdc_struc2vec_walk
Result:
_ids |
---|
["J","G","I","F"] |
["J","E","J"] |
["J","H","F","A"] |