UQL supports various values and types to represent data within the graph database. Understanding these values and types is essential for effective query construction and data manipulation.
Property Value Types
A property value type refers to the data type of the values of a property. Ultipa supports the following property value types:
Category | Type | Description |
---|---|---|
Numeric | int32 |
32-bit signed integer with a range from -2,147,483,648 to 2,147,483,647. |
uint32 |
32-bit unsigned integer with a range 0 to 4,294,967,295. | |
int64 |
64-bit signed integer with a range from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. | |
uint64 |
64-bit unsigned integer with a range from 0 to 18,446,744,073,709,551,615. | |
float |
32-bit single-precision floating-point number with 6 to 7 significant digits (integer and fractional parts, excl. the decimal point). | |
double |
64-bit double-precision floating-point number with 15 to 16 significant digits (integer and fractional parts, excl. the decimal point). | |
decimal |
Numbers can have a specified precision (1–65) and scale (0–30). For example, decimal(10,4) represents a decimal number with a precision of 10 (total digits, excluding the decimal point) and a scale of 4 (digits after the decimal point). |
|
Textual | string |
A sequence of characters with a maximum length of 60,000 bytes. This is the default value type when creating a property. |
text |
A sequence of characters with no length limitation. | |
Temporal | datetime |
Date and time value with a range from 1000-01-01 00:00:00.000000 to 9999-12-31 23:59:59.499999, stored as uint64 . |
timestamp |
A specific point in time, measured in seconds since 1970-01-01 00:00:00 UTC, stored as uint32 .Note: If a value is assigned in date and time format, it is converted to a timestamp based on the local timezone or the timezone set via the SDK's RequestConfig . Similarly, when a timestamp is displayed in date and time format, it is also converted based on the local timezone or the timezone set via the SDK's RequestConfig . |
|
Boolean | bool |
Represents two possible values:
|
Spatial | point |
A two-dimensional geographical coordinate that indicate a specific position; the coordinate values are stored as double . |
List | <subType>[] |
An ordered collection of elements of the specified subtype, supporting all of the above types except bool . |
Set | set(<subType>) |
An ordered collection of distinct elements of the specified subtype, supporting all of the above types except bool . |
Constructed Value Types
A constructed value type is a data type comprising composite elements. UQL supports the following constructed type:
Type | Description |
---|---|
list |
An ordered homogenous or heterogeneous collection of elements. |
Result Types
A result type refers to the data type of the values returned by a query. Ultipa defines the following result types.
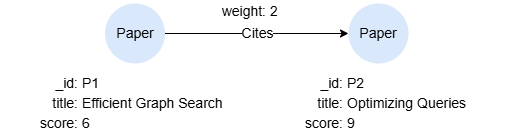
RESULT_TYPE_NODE
This query returns all the information of @Paper
nodes:
find().nodes({@Paper}) as n
return n{*}
Data structure of n
:
{
"data": [
{
"id": "P2",
"uuid": "8718971077612535835",
"schema": "Paper",
"values": {
"title": "Optimizing Queries",
"score": 9
}
},
{
"id": "P1",
"uuid": "8791028671650463770",
"schema": "Paper",
"values": {
"title": "Efficient Graph Search",
"score": 6
}
}
],
"alias": "n",
"type": 2,
"type_desc": "RESULT_TYPE_NODE"
}
RESULT_TYPE_EDGE
This query returns all the information of outgoing @Cites
edges:
n().re({@Cites} as e).n()
return e{*}
Data structure of e
:
{
"data": [
{
"from": "P1",
"to": "P2",
"uuid": "1",
"from_uuid": "8791028671650463770",
"to_uuid": "8718971077612535835",
"schema": "Cites",
"values": {
"weight": 2
}
}
],
"alias": "e",
"type": 3,
"type_desc": "RESULT_TYPE_EDGE"
}
RESULT_TYPE_PATH
This query returns all the information of outgoing 1-step paths:
n().re().n() as p
return p{*}
Data structure of p
:
{
"data": [
{
"nodes": [
{
"id": "P1",
"uuid": "8791028671650463770",
"schema": "Paper",
"values": {
"title": "Efficient Graph Search",
"score": 6
}
},
{
"id": "P2",
"uuid": "8718971077612535835",
"schema": "Paper",
"values": {
"title": "Optimizing Queries",
"score": 9
}
}
],
"edges": [
{
"from": "P1",
"to": "P2",
"uuid": "1",
"from_uuid": "8791028671650463770",
"to_uuid": "8718971077612535835",
"schema": "Cites",
"values": {
"weight": 2
}
}
],
"length": 1
}
],
"alias": "p",
"type": 1,
"type_desc": "RESULT_TYPE_PATH"
}
RESULT_TYPE_ATTR
This query returns the title
property of @Paper
nodes:
find().nodes({@Paper}) as n
return n.title
Data structure of n.title
:
{
"data": {
"alias": "n.title",
"type": 4,
"type_desc": "RESULT_TYPE_ATTR",
"values": [
"Optimizing Queries",
"Efficient Graph Search"
]
},
"alias": "n.title",
"type": 4,
"type_desc": "RESULT_TYPE_ATTR"
}
RESULT_TYPE_TABLE
This query returns a table:
find().nodes({@Paper}) as n
return table(n.title, n.score) as table
Data structure of table
:
{
"data": {
"name": "table",
"alias": "table",
"headers": [
"n.title",
"n.score"
],
"rows": [
[
"Optimizing Queries",
"9"
],
[
"Efficient Graph Search",
"6"
]
]
},
"alias": "table",
"type": 5,
"type_desc": "RESULT_TYPE_TABLE"
}
Null Value
The null
value is a special value available in all nullable types. Any non-null value is a material value.
Null Scenarios
The null
values can arise in various contexts, including:
- Default Assignment: When nodes or edges are inserted, nullable properties that lack specified values automatically receive
null
. - Explicit Null Specification: During node or edge insertion, nullable properties can be intentionally set to
null
. - Value Removal: Removing a property’s value sets it to
null
. - New Property Assignment: When adding a new property to a schema, any existing nodes or edges with that schema are assigned
null
for the new property by default. - Nonexistent Property References: Referencing a property that does not exist returns
null
. - Optional Matching: When the
OPTIONAL
keyword is used with statements likefind()
andkhop()
, if no result is found, the clause yieldsnull
instead of empty return.
Null in Comparisons
The null
value is not comparable to any other value due to its inherently unknown nature. Consequently, comparisons involving null
using normal operators such as =
or >
do not typically yield true or false but rather an unknown result, also represented by null
.
Example | Result |
---|---|
RETURN null = null |
null |
RETURN null > 3 |
null |
RETURN [1,null,2] <> [1,null,2] |
null |
RETURN 3 IN [1,null,2] |
null |
RETURN null IN [1,2] |
null |
RETURN null IN [] |
0 |
Comparisons involving null
require special handling with null predicates (IS NULL
and IS NOT NULL
).
Example | Result |
---|---|
RETURN null IS NULL |
1 |
RETURN null IS NOT NULL |
0 |
Null Treatments
The null
values receive special treatment in some contexts. For instance:
- Aggregate functions typically ignore
null
values. - The
GROUP BY
clause groups allnull
values together.