This section introduces methods for the insertion of nodes and edges.
Methods | Mechanism | Use Case | Note |
---|---|---|---|
insertNodes() insertEdges() |
Uses UQL under the hood. | Inserts a small number of nodes or edges. | |
insertNodesBatchBySchema() insertEdgesBatchBySchema() |
Uses gRPC to send data directly to the server. | Inserts large volumes of nodes or edges of the same schema. | The property values must be assigned using Python data types that correspond to the Ultipa supported property types (see Property Type Mapping). |
insertNodesBatchAuto() insertEdgesBatchAuto() |
Inserts large volumes of nodes or edges of different schemas. |
Property Type Mapping
The mappings between Ultipa property types and Python data types are as follows:
Ultipa Property Type |
Python Data Type |
Examples |
---|---|---|
INT32 , UINT32 , INT64 , UINT64 |
int |
18 |
FLOAT , DOUBLE |
float |
170.5 |
DECIMAL |
Decimal |
65.32 |
STRING , TEXT |
str |
"John Doe" |
DATETIME |
str [1] |
"1993-05-06" |
TIMESTAMP |
str [1], int |
"1993-05-06" , 1715169600 |
BOOL |
bool |
True , False |
POINT |
str |
"point({latitude: 132.1, longitude: -1.5})" |
LIST |
list |
["tennis", "violin"] |
SET |
set |
[2004, 3025, 1025] |
[1] Supported date string formats include [YY]YY-MM-DD HH:MM:SS
, [YY]YY-MM-DD HH:MM:SSZ
, [YY]YY-MM-DDTHH:MM:SSZ
, [YY]YY-MM-DDTHH:MM:SSXX
, [YY]YY-MM-DDTHH:MM:SSXXX
, [YY]YY-MM-DD HH:MM:SS.SSS
and their variations.
Example Graph Structure
The examples in this section demonstrate the insertion and deletion of nodes and edges in a graph based on the following schema and property definitions:
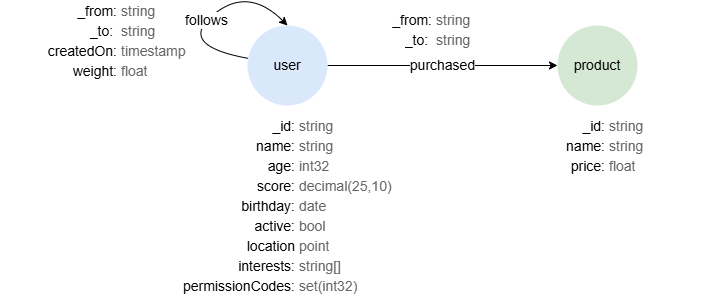
To create this graph structure, see the example provided here.
insertNodes()
Inserts nodes to a schema in the graph.
Parameters
nodes: List[Node]
: The list of nodes to be inserted.schemaName: str
: Schema name.config: InsertRequestConfig
(Optional): Request configuration.
Returns
Response
: Response of the request.
# Inserts two 'user' nodes into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
nodes = [
Node(id="U1", values={
"name": "Alice",
"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": 0,
"location": "point({latitude: 132.1, longitude: -1.5})",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", values={
"name": "Bob"
})
]
response = Conn.insertNodes(nodes, "user", insertRequestConfig)
if response.status.code.name == "SUCCESS":
print(response.status.code.name)
else:
print(response.status.message)
SUCCESS
insertEdges()
Inserts edges to a schema in the graph.
Parameters
edges: List[Edge]
: The list of edges to be inserted; the attributesfromId
andtoId
of eachEdge
are mandatory.schemaName: str
: Schema name.config: InsertRequestConfig
(Optional): Request configuration.
Returns
Response
: Response of the request.
# Inserts two 'follows' edges to the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
edges = [
Edge(fromId="U1", toId="U2", values={"createdOn": "2024-5-6", "weight": 3.2}),
Edge(fromId="U2", toId="U1", values={"createdOn": 1715169600})
]
response = Conn.insertEdges(edges, "follows", insertRequestConfig)
if response.status.code.name == "SUCCESS":
print(response.status.code.name)
else:
print(response.status.message)
SUCCESS
insertNodesBatchBySchema()
Inserts nodes to a schema in the graph through gRPC. This method is optimized for bulk insertion.
Parameters
schema: Schema
: The target schema; the attributename
is mandatory,properties
includes partial or all properties defined for the corresponding schema in the graph.nodes: List<Node>
: The list of nodes to be inserted; the attributevalues
of eachNode
must have the same structure withSchema.properties
.config: InsertRequestConfig
(Optional): Request configuration.
Returns
InsertResponse
: Response of the insertion request.
# Inserts two 'user' nodes into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
schema = Schema(
name="user",
properties=[
Property(name="name", type=UltipaPropertyType.STRING),
#Property(name="age", type=UltipaPropertyType.INT32),
Property(name="score", type=UltipaPropertyType.DECIMAL, decimalExtra=DecimalExtra(25, 10)),
Property(name="birthday", type=UltipaPropertyType.DATETIME),
Property(name="active", type=UltipaPropertyType.BOOL),
Property(name="location", type=UltipaPropertyType.POINT),
Property(name="interests", type=UltipaPropertyType.LIST, subType=[UltipaPropertyType.STRING]),
Property(name="permissionCodes", type=UltipaPropertyType.SET, subType=[UltipaPropertyType.INT32])
]
)
nodes = [
Node(id="U1", values={
"name": "Alice",
#"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": 0,
"location": "point({latitude: 132.1, longitude: -1.5})",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", values={
"name": "Bob",
#"age": None,
"score": None,
"birthday": None,
"active": None,
"location": None,
"interests": None,
"permissionCodes": None
})
]
insertResponse = Conn.insertNodesBatchBySchema(schema, nodes, insertRequestConfig)
if insertResponse.errorItems:
print("Error items:", insertResponse.errorItems)
else:
print("All nodes inserted successfully")
All nodes inserted successfully
insertEdgesBatchBySchema()
Inserts edges to a schema in the graph through gRPC. This method is optimized for bulk insertion.
Parameters
schema: Schema
: The target schema; the attributename
is mandatory,properties
includes partial or all properties defined for the corresponding schema in the graph.edges: List[Edge]
: The list of edges to be inserted; the attributesfrom
andto
of eachEdge
are mandatory,values
must have the same structure withSchema.properties
.config: InsertRequestConfig
(Optional): Request configuration.
Returns
InsertResponse
: Response of the insertion request.
# Inserts two 'follows' edges into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
schema = Schema(
name="follows",
properties=[
#Property(name="createdOn", type=UltipaPropertyType.TIMESTAMP),
Property(name="weight", type=UltipaPropertyType.FLOAT)
]
)
edges = [
Edge(fromId="U1", toId="U2", values={
#"createdOn": "2024-5-6",
"weight": 3.2
}),
Edge(fromId="U2", toId="U1", values={
#"createdOn": 1715169600,
"weight": None
})
]
insertResponse = Conn.insertEdgesBatchBySchema(schema, edges, insertRequestConfig)
if insertResponse.errorItems:
print("Error items:", insertResponse.errorItems)
else:
print("All edges inserted successfully")
All edges inserted successfully
insertNodesBatchAuto()
Inserts nodes to one or multipe schemas in the graph through gRPC. This method is optimized for bulk insertion.
Parameters
nodes: List[Node]
: The list of nodes to be inserted; the attributeschema
of eachNode
are mandatory, thevalues
of all nodes must have the same structure and include partial or all properties defined for the corresponding schema in the graph.config: InsertRequestConfig
(Optional): Request configuration.
Returns
Dict[str,InsertResponse]
: The schema name, and response of the insertion request.
# Inserts two 'user' nodes and a 'product' node into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
nodes = [
Node(id="U1", schema="user", values={
"name": "Alice",
#"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": True,
"location": "point({latitude: 132.1, longitude: -1.5})",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", schema="user", values={
"name": "Bob",
#"age": None,
"score": None,
"birthday": None,
"active": None,
"location": None,
"interests": None,
"permissionCodes": None
}),
Node(schema="product", values={
"name": "Wireless Earbud",
"price": 93.2
})
]
result = Conn.insertNodesBatchAuto(nodes, insertRequestConfig)
for schemaName, insertResponse in result.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "nodes:", insertResponse.errorItems)
else:
print("All", schemaName, "nodes inserted successfully")
All user nodes inserted successfully
All product nodes inserted successfully
insertEdgesBatchAuto()
Inserts edges to one or multipe schemas in the graph through gRPC. This method is optimized for bulk insertion.
Parameters
edges: List[Edge]
: The list of edges to be inserted; the attributesschema
,fromId
, andtoId
are mandatory, thevalues
of all edges must have the same structure and include partial or all properties defined for the corresponding schema in the graph.config: InsertRequestConfig
(Optional): Request configuration.
Returns
Dict[str,InsertResponse]
: The schema name, and response of the insertion request.
# Inserts two 'follows' edges and a 'purchased' edge into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
edges = [
Edge(schema="follows", fromId="U1", toId="U2", values={"createdOn": "2024-05-06", "weight": 3.2}),
Edge(schema="follows", fromId="U2", toId="U1", values={"createdOn": 1715169600, "weight": None}),
Edge(schema="purchased", fromId="U2", toId="67da1ae90000020020000013", values={})
]
result = Conn.insertEdgesBatchAuto(edges, insertRequestConfig)
for schemaName, insertResponse in result.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "edges:", insertResponse.errorItems)
else:
print("All", schemaName, "edges inserted successfully")
All follows edges inserted successfully
All purchased edges inserted successfully
Full Example
from ultipa import UltipaConfig, Connection, InsertRequestConfig, Node
ultipaConfig = UltipaConfig()
# URI example: ultipaConfig.hosts = ["mqj4zouys.us-east-1.cloud.ultipa.com:60010"]
ultipaConfig.hosts = ["192.168.1.85:60061", "192.168.1.87:60061", "192.168.1.88:60061"]
ultipaConfig.username = "<username>"
ultipaConfig.password = "<password>"
Conn = Connection.NewConnection(defaultConfig=ultipaConfig)
# Inserts two 'user' nodes, a 'product' node, two 'follows' edges, and a 'purchased' edge into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
nodes = [
Node(id="U1", schema="user", values={
"name": "Alice",
#"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": True,
"location": "point({latitude: 132.1, longitude: -1.5})",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", schema="user", values={
"name": "Bob",
#"age": None,
"score": None,
"birthday": None,
"active": None,
"location": None,
"interests": None,
"permissionCodes": None
}),
Node(id="P1", schema="product", values={
"name": "Wireless Earbud",
"price": 93.2
})
]
edges = [
Edge(schema="follows", fromId="U1", toId="U2", values={"createdOn": "2024-05-06", "weight": 3.2}),
Edge(schema="follows", fromId="U2", toId="U1", values={"createdOn": 1715169600, "weight": None}),
Edge(schema="purchased", fromId="U2", toId="P1", values={})
]
result_n = Conn.insertNodesBatchAuto(nodes, insertRequestConfig)
for schemaName, insertResponse in result_n.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "nodes:", insertResponse.errorItems)
else:
print("All", schemaName, "nodes inserted successfully")
result_e = Conn.insertEdgesBatchAuto(edges, insertRequestConfig)
for schemaName, insertResponse in result_e.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "edges:", insertResponse.errorItems)
else:
print("All", schemaName, "edges inserted successfully")